The moment we all in the Tezos community have been waiting for so long has now come. We are releasing Alpha support for decentralized application interaction in Thanos Wallet.
The whole idea was to re-create the most familiar UX and easy-to-understand UI for everybody who has previously used DApps on other blockchains.
We believe that easy-to-use DApps is one of the most expected features for the Tezos community and it should provide a great boost to the development and user experience across the whole ecosystem.
How to enable DApps for your wallet
The feature itself is still quite experimental and only available after enabling it in the settings under the “DApps” section.
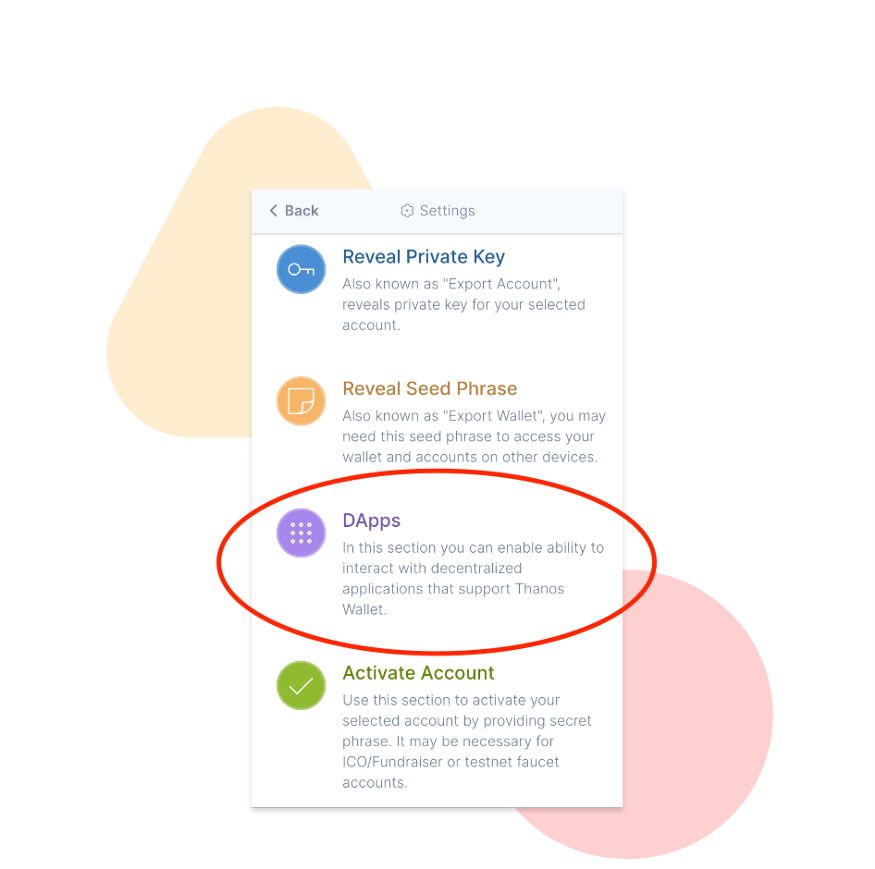
You have to click the checkmark on the DApp page to allow DApps webpages to access your wallet instance. It’s a simple security measure to prevent any potential abuse until the feature is properly tested and audited.
After completion of this step, you’re good to dive into the DApp development part!
Writing a simple Counter DApp
The following piece of code is a simple Counter contract that stores value on blockchain and allows anybody to change it via increment and decrement entry points:
# Counter - Example for illustrative purposes only.
import smartpy as sp
class Counter(sp.Contract):
def __init__(self, initialValue = 0):
self.init(storedValue = initialValue)
@sp.entry_point
def increment(self, params):
self.data.storedValue += params.value
@sp.entry_point
def decrement(self, params):
self.data.storedValue -= params.value
The above contract can be easily deployed using Truffle Suite for Tezos (it’s also still experimental, but works just fine).
You can find a full guide on deployment via the following link: https://www.trufflesuite.com/docs/tezos/truffle/quickstart
To interact with Tezos blockchain using Thanos Wallet, you should install two required dependencies:
yarn add @taquito/taquito@^6.3.0-wallet.3 @thanos-wallet/dapp
The following piece of code shows how easy it is to call smart-contract methods and get account balance using our API:
import { ThanosWallet } from "@thanos-wallet/dapp";
(async () => {
try {
const available = await ThanosWallet.isAvailable();
if (!available) {
throw new Error("Thanos Wallet not installed");
}
const wallet = new ThanosWallet("My Super DApp");
await wallet.connect("carthagenet");
const tezos = wallet.toTezos();
const accountPkh = await tezos.wallet.pkh();
const accountBalance = await tezos.tz.getBalance(accountPkh);
console.info(`address: ${accountPkh}, balance: ${accountBalance}`);
const counter = await tezos.wallet.at(
"KT1DjYkruvfujfKw6nLYafArqKufcwHuKXvT"
);
const operation = await counter.methods.increment(1).send();
await operation.confirmation();
const counterValue = await counter.storage();
console.info(`count: ${counterValue}`);
} catch (err) {
console.error(err);
}
})();
On the await wallet.connect()
step the wallet will show a confirmation pop-up prompting a user to allow interaction between the wallet and the DApp webpage.
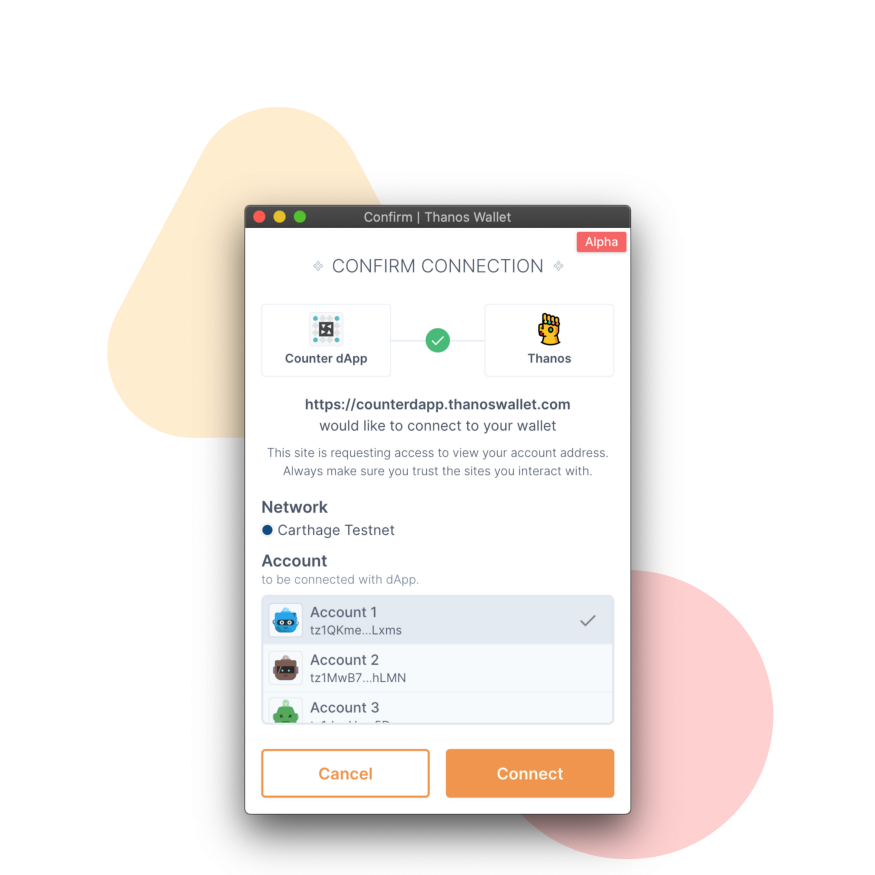
When the JavaScript code invokes a method on a contract or tries to perform any operation on the blockchain, the operation confirmation pop-up would appear allowing a user to either accept or decline it.
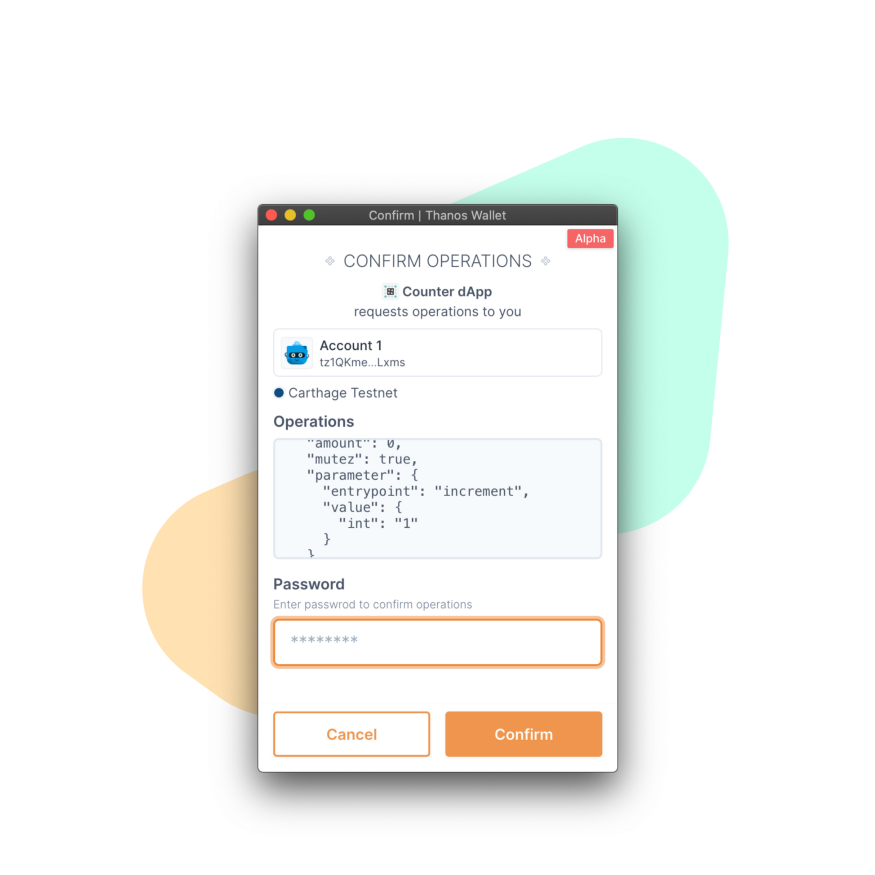
After pressing the confirm button the operation will be propagated to the blockchain network and by calling await operation.confirmation()
you’re able to wait until the operation is successfully confirmed.
That’s it! You can learn more about the API we provide on the @thanos-wallet/dapp repo page
Resources
If you want to learn more or try it yourself you can take a look at the code in our example Github repo:
https://github.com/madfish-solutions/counter-dapp
Demo of the deployed DApp:

Important Notes
It still Alpha Open-Source software, which means API is likely to change. Also, there’s a chance that it may contain bugs and vulnerabilities, which may lead to loss of real funds on mainnet — please, use it at your own risk.
Happy testing!